Wrapping up
This is an optional step that takes what we’ve built so far and optimizes the app for a production environment.
Preview
A preview of what you’ll build (visually no different, but built for production):
Fork, clone and branch
This tutorial has an accompanying GitHub repository called carbon-tutorial-web-components that we’ll use as a starting point for each step. If you haven’t forked and cloned that repository yet, and haven’t added the upstream remote, go ahead and do so by following the step 1 instructions.
Branch
With your repository all set up, let’s check out the branch for this tutorial step’s starting point.
git fetch upstreamgit checkout -b step-5 upstream/step-5
Build and start app
Install the app’s dependencies (in case you’re starting fresh in your current directory and not continuing from the previous step):
pnpm i
Then, start the app:
pnpm dev
You should see something similar to where the previous step left off.
Linting
Stylelint
While the Carbon component styles are encapsulated in the components there we have written some SCSS ourselves it would great if we could check we are applying Carbon as expected.
For more information on Stylelint visit stylelint.io.
Add Stylelint, a Carbon plugin as development dependencies.
pnpm add -D stylelint stylelint-plugin-carbon-tokens
In
package.json
"lint:style": "pnpm stylelint '**/*.scss'",
In the root folder create a file called
.stylelintrc.json
{"extends": ["stylelint-plugin-carbon-tokens/config/recommended"],"plugins": ["stylelint-plugin-carbon-tokens"],"reportNeedlessDisables": true,"reportInvalidScopeDisables": true,"reportDescriptionlessDisables": true}
At the command line you can now run the script
lint:style
font-weight
$pnpm run lint:style> carbon-tutorial-web-components@0.0.0 lint:style /Users/leechase/Source/carbon/carbon-tutorial-web-components> pnpm stylelint '**/*.scss'style.scss158:16 âś– Expected carbon type token, mixin or function for carbon/type-use"font-weight" found "600".
If you open the file you will see that
600
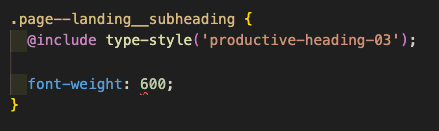
Hovering over it you will see a popup explanation.
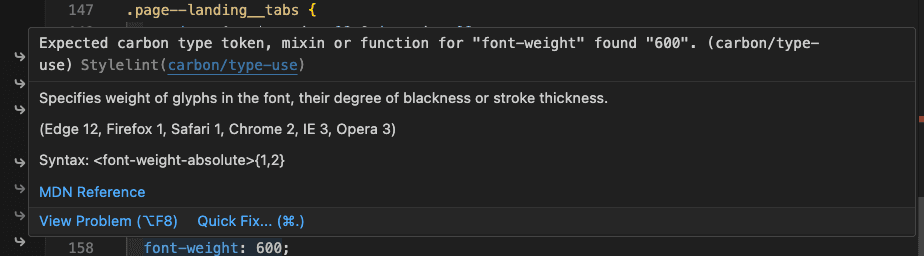
Change
600
font-weight('semibold')
It is recommended that you use a more extensive rule set for stylelint. This can help keep code consistent and auto fix many potential issues.
If for instance you were to adopt the following configuration it would check for a number of important issues such as accessiblity, right to left, and even consistency patterns like nesting depty and CSS class selector pattern.
Even better the small number of issues that it raises, regarding use of logical properties, can be auto fixed bringing your CSS up to date.
{"extends": ["stylelint-config-standard-scss","stylelint-plugin-carbon-tokens/config/recommended"],"plugins": ["@double-great/stylelint-a11y","stylelint-use-logical-spec","stylelint-plugin-carbon-tokens"
Don’t forget to add these packages if you want to try out this config.
pnpm add -D @double-great/stylelint-a11y stylelint-config-standard-scss stylelint-use-logical stylelint-use-logical-spec
Running
pnpm run lint:style --fix
ESLint & Prettier
In addition to linting styles, it is recommended that you also check your script for dev time errors and consitency.
For more information on ESLint visit eslint.org.
For more information on Prettier visit prettier.io.
NOTE: If you are using a reactive library then there may well be configurations better suited to your purposes.
ESLint checks your code for potential bugs, Prettier ensures your whole teams code uses the same formatting rules. Config for these two is often added at the same time, the package
eslint-config-prettier
Create a file called
.prettierrc
{"bracketSameLine": true,"bracketSpacing": true,"printWidth": 120,"semi": true,"singleQuote": true,"tabWidth": 2,"trailingComma": "es5","proseWrap": "always"
Then add a file called
eslint.config.js
import js from '@eslint/js';import eslintConfigPrettier from 'eslint-config-prettier';import globals from 'globals';export default [{languageOptions: {ecmaVersion: 2024,globals: {
Add the following dev dependencies
pnpm add -D eslint eslint-config-prettier prettier globals
Two more scripts for
package.json
"lint:es": "eslint . main.js --report-unused-disable-directives --max-warnings 0","lint:format": "prettier ./**/*.{js,jsx,ts,tsx,md,mdx,scss} --write --ignore-unknown --no-error-on-unmatched-pattern --log-level warn",
Running these scripts will, not at the time of writing highlight any ESLint issues. If it does in future it may be possible to auto fix them.
It will almost certainly refomat the code compared to your chosen editors preferences.
Spell checkers
If we all had perfect spelling and typing then maybe a spell checker is not needed. However, given that the word
typo
A spell checker ensures that
typo
For more information on cspell visit cspell.org.
Add
cspell
pnpm add -D cspell
There are many config options, including the ability to create and share custom dictionaries so your brand, pet name or other desirable creations do not get flagged up as spelling errors.
Here we will add the following config file
cspell.json
{"version": "0.1","language": "en","dictionaries": [],"dictionaryDefinitions": [],"enabledLanguageIds": ["css","git-commit","html",
Add this spelling script to
package.json
"lint:spell": "cspell lint --quiet \"**/*.{js,css,scss,md}\"",
Running this script will find a number of spellings
cspell
In our style file for instance it will highlight our image name
illo
We can also see in our IDE that this is highlighted with a blue wiggly underline.
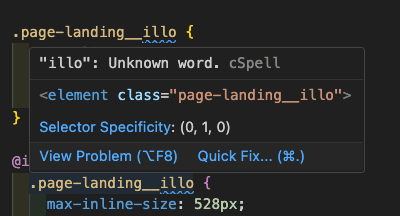
There are a number of ways to fix this. As this is not a spelling mistake we will choose
Add: "illo" to config:...
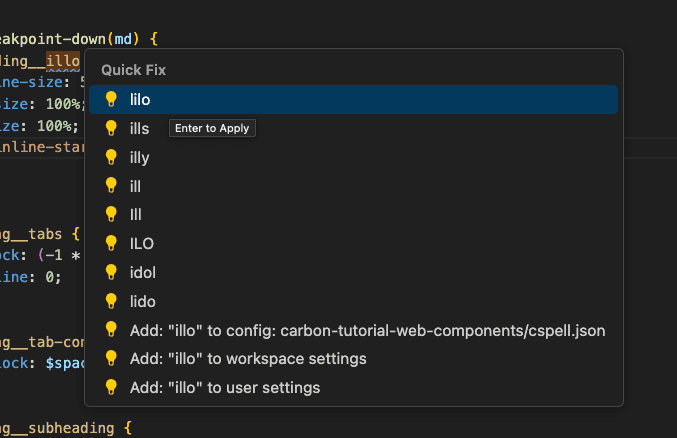
If you view the
cspell.json
Deplying to gh-pages
GitHub packages can use a branch called
gh-pages
Update and/or add
name
homepage
pacakge.json
"name": "carbon-tutorial-web-components","homepage": "https://github.com/[YOUR-ORG-OR-NAME]/[REPO-NAME]",
Back to
package.json
pnpm add -D gh-pages
Next to run the deployment we will add two scripts to
package.json
"predeploy": "npm run build","deploy": "gh-pages -d dist",
Way back in step 1 you creted a fork of the tutorial repo. It is this URL you use for the projects homepage.
Next you will need a
vite.config.js
index.html
repositories.html
base
If a personal GitHub it is likely your vite config will look like this:
export default {base: '/[REPO-NAME]/',build: {rollupOptions: {input: {landing: fileURLToPath(new URL('./index.html', import.meta.url)),repositories: fileURLToPath(new URL('./repositories.html', import.meta.url)),
If part of an organisation use
base: '/'
After running the deploy script you will have a publicly visible page. You can locate the page by browsing to the settings page of your GitHub repository. At the time of writing the URL shown in the
Pages
For ease of access:
- Select the tab/pageCode
- Click on the cog (right side of page) to the right of to view the repository details.About
- Check the and save changes. The GitHub pages URL should now appear on the landing page of your repository.Use your GitHub Pages website
Debugging your deploy
Build
Run a build and makes sure your assets are all included in the
dist
pnpm build
This should include the contents of public, the two html files, CSS and Javascript. If something is missing check your Vite config and
package.json
####Â Deploy
Deploy your application with a base value of
/
Open the deveveloper tools and view the console. Any missing assets should cause messages such as the following to be displayed.

In this case
- GitHub pages location https://[ACCOUNT].github.io/[REPO-NAME]
- Asset location https://[ACCOUNT].github.io/[ASSET]
The fix would be to change your base setting to
/[REPO-NAME]/
Using the CDN
Lastly we’ve been using
main.js
node_modules
Remove the component imports from
main.js
import '@carbon/web-components/es/components/button/button.js';import '@carbon/web-components/es/components/ui-shell/index';import '@carbon/web-components/es/components/checkbox/index';import '@carbon/web-components/es/components/content-switcher/index';import '@carbon/web-components/es/components/skip-to-content/index.js';
Remove the component imports from
landing.js
import '@carbon/web-components/es/components/breadcrumb/index';import '@carbon/web-components/es/components/tabs/index';
Remove the component imports from
repos.js
import '@carbon/web-components/es/components/data-table/index.js';import '@carbon/web-components/es/components/link/index';import '@carbon/web-components/es/components/pagination/index';
In the
head
index.html
<scripttype="module"src="https://1.www.s81c.com/common/carbon/web-components/version/v2.17.0/button.min.js"></script><scripttype="module"src="https://1.www.s81c.com/common/carbon/web-components/version/v2.17.0/ui-shell.min.js"></script><scripttype="module"src="https://1.www.s81c.com/common/carbon/web-components/version/v2.17.0/checkbox.min.js"></script>
We also need to update
repositories.html
<scripttype="module"src="https://1.www.s81c.com/common/carbon/web-components/version/v2.17.0/ui-shell.min.js"></script><scripttype="module"src="https://1.www.s81c.com/common/carbon/web-components/version/v2.17.0/checkbox.min.js"></script><scripttype="module"src="https://1.www.s81c.com/common/carbon/web-components/version/v2.17.0/content-switcher.min.js"></script>
If you run the application again you are running with no dependency on
node_modules/@carbon/web-components
Push to GitHub
Just one more push to save your completion of step 5.
Git commit and push
First, stage and commit all of your changes:
git add --all && git commit -m "feat(tutorial): complete step 5"
Then, push to your repository:
git push -u origin step-5
Step 5 complete branch
That is it you are done. If you want to review the completed step 5 you can do so by checking out
git fetch upstreamgit checkout -b step-5 upstream/step-5-complete